You can use the transpose method to change the row vector into a column vector.
>> A = [1 9 3 7 3] >> B = A' B = 1 9 3 7 3
2. How to convert a column vector into the row vector?
Same as above use transpose method to change the column vector into a row vector.>> C = B' C = 1 9 3 7 3The result on the MATLAB window:
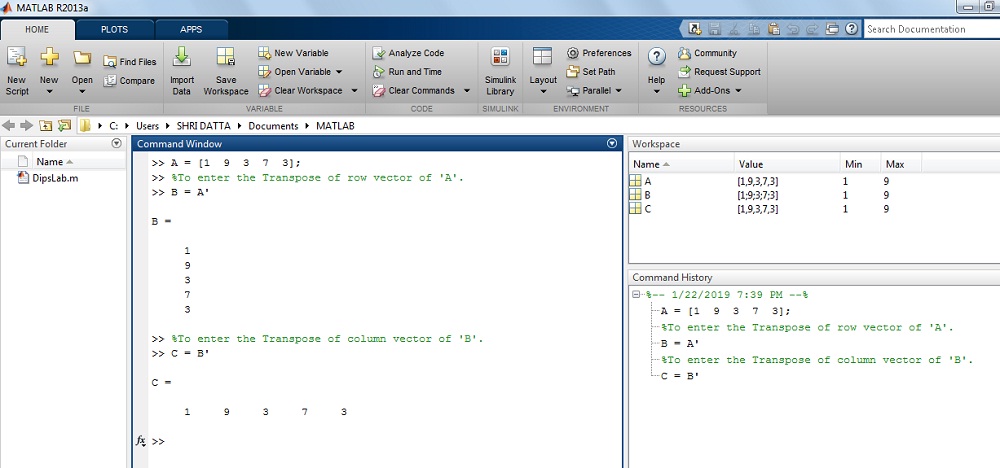
MATHEMATICAL OPERATIONS ON VECTOR
In the last post, I have explained the multiple Mathematical functions and their syntax in MATLAB. These mathematical functions and their syntax are useful in the vector operation as well.1. Arithmetic Operation of Vector:
The basic arithmetic operation consists of the addition (+), subtraction (-), multiplication (*), etc. Here, I am doing some basic mathematical operations. For Example:>> A = [7 5 33 61]; >> B =[21 3 1 4]; >> Addition of two vector A and B >> X = (A + B) X = 28 8 34 65 >> Substraction of two vector A and B >> Y = (A-B) Y = -14 2 32 57 >> Mutliplication of vector >> z=(4*y) z = -56 8 128 228The result on MATLAB display:
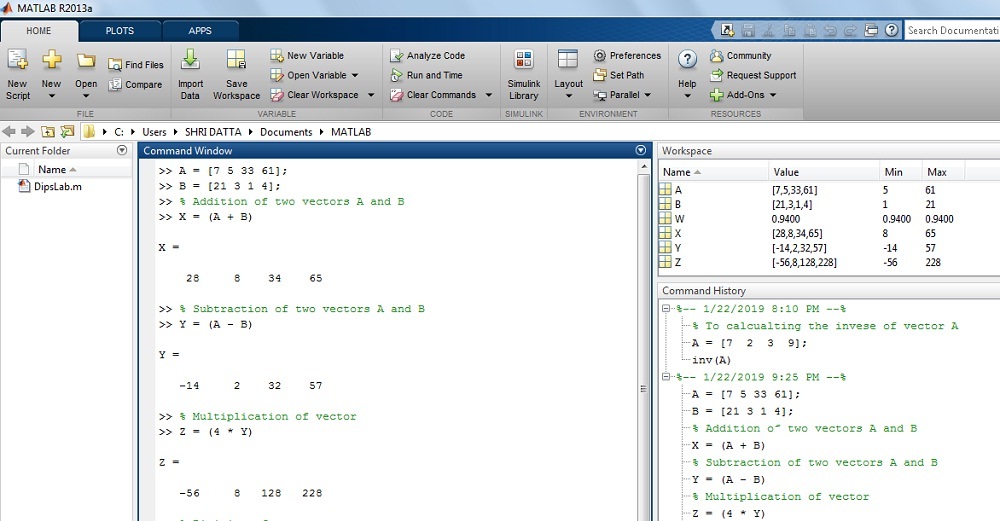
2. Length of Vector:
Length function is useful for calculating the number of elements in the vector. In the MATLAB, there is a length method. Example: How to calculate the length of the vector in MATLAB? Here is a syntax.>> X = [2 4 1 7 8]; >> length(X) = 5The Result on MATLAB display:
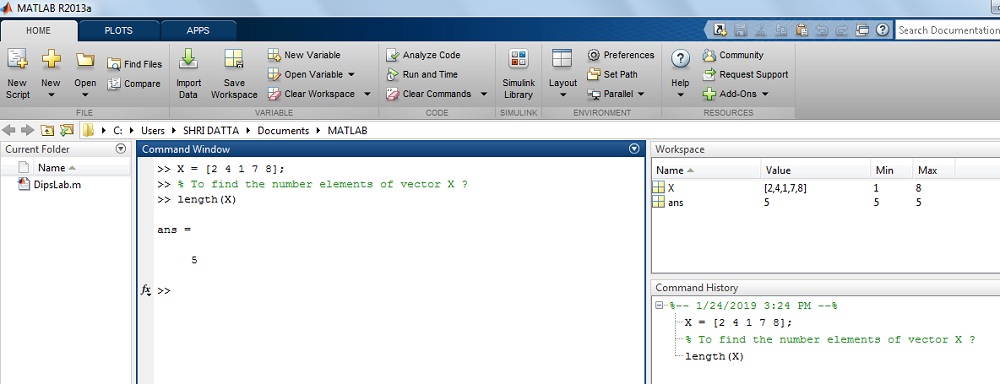